Almost after breaking my resolution of frequent blogging, here I present to you a post about the last Codechef Contest I did the DPS Dwarka INOI practice.
The contest was fairly easy, I don't think it kind of lived up to the name of the INOI practice. But I think it was a nice contest, and somehow I ended up solving all the 6 problems.
So here's something straight from the horse's mouth (wait did I just called myself a horse ? ummm...)
Problem 1 :- Chef and Powers of 2
So here we have another codegolf problem and almost immediately the word that pops into my head is "Python"(that mystical language used by the ancients to treat all maladies) that can be used to express my ideas in the most consize manner as possible.
The problem basically states to decompose an even number as a sum of distinct powers of 2 in increasing order.
My first approch :-
Take the number convert it to its binary equivalent string and if the character at i'th postion is '1' print 2^i but you know what ? It was TOO LONG 100 characters OMG unsuitable for codegolf and it fetched me just 0.34 points. So back to the drawing board.
Realized that why do I have to convert the number to a binary string when it's stored as a binary number in memory.
So cutting down some unnecessary code gives.
So something better but wait a minute bitshifting ??? it's unecessary it costs me 2 characters while mutiplication will make it just 1.
Cute isn't it ? Wait I can do better. the last step was to remove indents and that made me learn the inline if statement of python
The final version
now removing the indents makes it kind of ugly from python's point but for codegolf its just fine so here I was with 0.708 points on the board. cool now moving onto the next problem
Problem 2:- Chef and Fibonacci
So the problem states that :-
given two numbers A,B find the C'th fibonacci number where C=gcd(A,B) and since the number can be pretty large print it modulo 10^9+7 (pretty standard one).
Since the constraints are fairly large so here's the place to put your matrix exponentiation skills to action.
So as learnt from e-maxx the following equations are great to observe the behaviour of Fibonacci series
![\ Pmatrix {F_ {n-2} & F_ {n-1} \ cr} \ cdot \ pmatri [...]](http://e-maxx.ru/tex2png/cache/dae8aa23f9a9ab8ca68d653890d7200b.png)
So if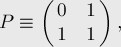
Then![\ Pmatrix {F_0 & F_1 \ cr} \ cdot P ^ n = \ pmatrix {[...]](http://e-maxx.ru/tex2png/cache/5f31c53e54bed1cce6c326dce75c3157.png)
Now time for some code. It's nice to observe that matrix exponentiation is just the same as binary exponentiation establising the the essence of symmetry between the mathematical entities.
modular raises them to power and rest is nothing but python's awesomeness.
So here was another problem in the bag.
Problem 3:- Chef and mountains
Problem statement:- Given an array and n queries of two numbers A and B check that the max value in range [A,B] is greater than value at index A or not. Another standard problem , R.M.Q.
nothing special about the implementation either.
Nothing special, just initialize the Segment tree and start making queries.
Not to mention that got 6 WA's due to an undetected bug.
Problem 4:- Chef and moon
The Problem asks to find all pairs of shortest paths from any source to destination and print "YES" if it's less than or equal to a given number k otherwize print "NO"
Take a look at the question and it just screams of Floyd-Warshall. So nothing special about it at all either, I was surprised at the fact that only 370 people solved it.
Problem 5:- Chef and array
Number of ways of partitionong an array in to 3 contiguous parts such that each part gets same sum.
Straight forward memoization.
1) Convert the array to it's prefix sum.
2) If sum of all elements is not divisible by 3 print 0.
3) Memoize for each index i how many elements in the remaining indices greater than i have value 2*(sum[n]/3)
4) for each index i where sum[i] == (sum[n]/3) add the memoized value to a counter.
The code will explain better I guess.
Problem 6:- Chef and confusing road
Tell you what Deja vu problem Unbearable Controversy of being. with relaxed constraints. My solution which got TLE on codeforces AC here on one go.
The procedure i followed was to take the any two nodes and find next successive members of the graph and take the intersection of both and add it to the count.
See you guys Later.
Adios.
The contest was fairly easy, I don't think it kind of lived up to the name of the INOI practice. But I think it was a nice contest, and somehow I ended up solving all the 6 problems.
So here's something straight from the horse's mouth (wait did I just called myself a horse ? ummm...)
Problem 1 :- Chef and Powers of 2
So here we have another codegolf problem and almost immediately the word that pops into my head is "Python"(that mystical language used by the ancients to treat all maladies) that can be used to express my ideas in the most consize manner as possible.
The problem basically states to decompose an even number as a sum of distinct powers of 2 in increasing order.
My first approch :-
- n=int(input())
- s="{0:b}".format(n)
- s=s[::-1] #reverses the string magically :P
- for i in xrange(len(s)):
- if s[i]=='1':
- print 2**(i),
Take the number convert it to its binary equivalent string and if the character at i'th postion is '1' print 2^i but you know what ? It was TOO LONG 100 characters OMG unsuitable for codegolf and it fetched me just 0.34 points. So back to the drawing board.
Realized that why do I have to convert the number to a binary string when it's stored as a binary number in memory.
So cutting down some unnecessary code gives.
- n=int(input());i=1
- while (1<<i)<=n:
- if ((1<<i)&n):
- print 1<<i,
- i+=1
So something better but wait a minute bitshifting ??? it's unecessary it costs me 2 characters while mutiplication will make it just 1.
- n,k=input(),2
- while k<=n:
- if k&n:print k,
- k*=2
Cute isn't it ? Wait I can do better. the last step was to remove indents and that made me learn the inline if statement of python
The final version
- n,k=input(),2
- while k<=n:print k if k&n else '',;k*=2
now removing the indents makes it kind of ugly from python's point but for codegolf its just fine so here I was with 0.708 points on the board. cool now moving onto the next problem
Problem 2:- Chef and Fibonacci
So the problem states that :-
given two numbers A,B find the C'th fibonacci number where C=gcd(A,B) and since the number can be pretty large print it modulo 10^9+7 (pretty standard one).
Since the constraints are fairly large so here's the place to put your matrix exponentiation skills to action.
So as learnt from e-maxx the following equations are great to observe the behaviour of Fibonacci series
![\ Pmatrix {F_ {n-2} & F_ {n-1} \ cr} \ cdot \ pmatri [...]](http://e-maxx.ru/tex2png/cache/dae8aa23f9a9ab8ca68d653890d7200b.png)
So if
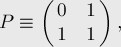
Then
![\ Pmatrix {F_0 & F_1 \ cr} \ cdot P ^ n = \ pmatrix {[...]](http://e-maxx.ru/tex2png/cache/5f31c53e54bed1cce6c326dce75c3157.png)
Now time for some code. It's nice to observe that matrix exponentiation is just the same as binary exponentiation establising the the essence of symmetry between the mathematical entities.
- from fractions import gcd
- basis=[[0,1],[1,1]]
- def multiply(m1,m2):
- one=(m1[0][0]*m2[0][0]+m1[0][1]*m2[1][0])%(10**9+7)
- two=(m1[0][0]*m2[0][1]+m1[0][1]*m2[1][1])%(10**9+7)
- three=(m1[1][0]*m2[0][0]+m1[1][1]*m2[1][0])%(10**9+7)
- four=(m1[1][0]*m2[0][1]+m1[1][1]*m2[1][1])%(10**9+7)
- return [[one,two],[three,four]]
- def modular(base,exponent):
- res=[[1,0],[0,1]]
- while exponent:
- if exponent%2==1:
- res=multiply(res,base)
- exponent-=1
- else:
- base=multiply(base,base)
- exponent>>=1
- return res
- t=int(input())
- while t>0:
- t-=1
- a,b=map(int,raw_input().split())
- c=gcd(a,b)
- l=basis
- ans=modular(l,c-1)
- print (ans[0][0]+ans[1][0])%(10**9+7)
modular raises them to power and rest is nothing but python's awesomeness.
So here was another problem in the bag.
Problem 3:- Chef and mountains
Problem statement:- Given an array and n queries of two numbers A and B check that the max value in range [A,B] is greater than value at index A or not. Another standard problem , R.M.Q.
nothing special about the implementation either.
Nothing special, just initialize the Segment tree and start making queries.
Not to mention that got 6 WA's due to an undetected bug.
- #include <cstring>
- #include <vector>
- #include <list>
- #include <map>
- #include <set>
- #include <deque>
- #include <stack>
- #include <bitset>
- #include <algorithm>
- #include <functional>
- #include <numeric>
- #include <utility>
- #include <sstream>
- #include <iostream>
- #include <iomanip>
- #include <cstdio>
- #include <cmath>
- #include <cstdlib>
- #include <ctime>
- #include <memory.h>
- using namespace std;
- #define FOR(i,a) for(int i = 0;i < a;i++)
- #define REP(i,a,b) for(int i = a;i < b;i++)
- #define vi vector<int>
- #define MAXN 5000010
- int ar[MAXN],M[MAXN];
- void init(int ar[MAXN],int M[MAXN],int node,int b,int e)
- {
- if(b==e)
- {
- M[node]=ar[e];
- return ;
- }
- init(ar,M,2*node+1,b,(b+e)/2);
- init(ar,M,2*node+2,(b+e)/2+1,e);
- M[node] = max(M[2*node+1] , M[2*node+2]);
- }
- int query(int ar[MAXN],int M[MAXN],int node,int b,int e,int i,int j)
- {
- if(i>e||j<b)//out of bounds
- {
- return -1;
- }
- if(b>=i&&e<=j)
- {
- return M[node];
- }
- int p1,p2;
- p1=query(ar,M,2*node+1,b,(b+e)/2,i,j);
- p2=query(ar,M,2*node+2,(b+e)/2+1,e,i,j);
- return max(p1,p2);
- }
- int main()
- {
- int n,q,a,b,c;
- scanf("%d",&n);
- FOR(i,n)
- {
- scanf("%d",&ar[i]);
- }
- init(ar,M,0,0,n-1);
- scanf("%d",&q);
- while(q--)
- {
- scanf("%d %d",&a,&b);
- if(b-a>=2)
- {
- c=query(ar,M,0 ,0,n-1,a,b-2);
- }
- else
- {
- printf("YES\n");
- continue;
- }
- if(c>ar[a-1])
- {
- printf("NO\n");
- }
- else
- {
- printf("YES\n");
- }
- }
- return 0;
- }
Problem 4:- Chef and moon
The Problem asks to find all pairs of shortest paths from any source to destination and print "YES" if it's less than or equal to a given number k otherwize print "NO"
Take a look at the question and it just screams of Floyd-Warshall. So nothing special about it at all either, I was surprised at the fact that only 370 people solved it.
- #include <cstring>
- #include <vector>
- #include <list>
- #include <map>
- #include <set>
- #include <deque>
- #include <stack>
- #include <bitset>
- #include <algorithm>
- #include <functional>
- #include <numeric>
- #include <utility>
- #include <sstream>
- #include <iostream>
- #include <iomanip>
- #include <cstdio>
- #include <cmath>
- #include <cstdlib>
- #include <ctime>
- #include <memory.h>
- using namespace std;
- #define FOR(i,a) for(int i = 0;i < a;i++)
- #define REP(i,a,b) for(int i = a;i < b;i++)
- #define vi vector<int>
- #define MAXN 1000000000
- int city[110][110];
- int main()
- {
- ios_base::sync_with_stdio(false);
- FOR(i,100)
- {
- fill(city[i],city[i]+100,MAXN);
- }
- int n,m,K,a,b,c;
- cin>>n>>m>>K;
- FOR(i,m)
- {
- cin>>a>>b>>c;
- city[a-1][b-1]=c;
- city[b-1][a-1]=c;
- }
- FOR(i,n)
- {
- city[i][i]=0;
- }
- for(int k=0;k<n;k++)
- {
- for(int i=0;i<n;i++)
- {
- for(int j=0;j<n;j++)
- {
- city[i][j]=min(city[i][j],city[i][k]+city[k][j]);
- }
- }
- }
- int q,u,v;
- cin>>q;
- while(q--)
- {
- cin>>u>>v;
- if(city[u-1][v-1]<=K)
- {
- cout<<"YES\n";
- }
- else
- {
- cout<<"NO\n";
- }
- }
- return 0;
- }
Problem 5:- Chef and array
Number of ways of partitionong an array in to 3 contiguous parts such that each part gets same sum.
Straight forward memoization.
1) Convert the array to it's prefix sum.
2) If sum of all elements is not divisible by 3 print 0.
3) Memoize for each index i how many elements in the remaining indices greater than i have value 2*(sum[n]/3)
4) for each index i where sum[i] == (sum[n]/3) add the memoized value to a counter.
The code will explain better I guess.
- #include <cstring>
- #include <vector>
- #include <list>
- #include <map>
- #include <set>
- #include <deque>
- #include <stack>
- #include <bitset>
- #include <algorithm>
- #include <functional>
- #include <numeric>
- #include <utility>
- #include <sstream>
- #include <iostream>
- #include <iomanip>
- #include <cstdio>
- #include <cmath>
- #include <cstdlib>
- #include <ctime>
- #include <memory.h>
- using namespace std;
- #define FOR(i,a) for(int i = 0;i < a;i++)
- #define REP(i,a,b) for(int i = a;i < b;i++)
- #define vi vector<int>
- #define MAXN 1000000
- #define LL long long
- LL ar[MAXN];
- int tr[MAXN];
- int main()
- {
- int n;
- fill(tr,tr+MAXN,0);
- scanf("%d",&n);
- FOR(i,n)
- {
- scanf("%lld",&ar[i]);
- if(i!=0)
- {
- ar[i]+=ar[i-1];
- }
- }
- if(ar[n-1]%3!=0)
- {
- printf("0\n");
- return 0;
- }
- LL tw=2*(ar[n-1]/3);
- LL on=(ar[n-1]/3);
- for(int i=n-3;i>=0;i--)
- {
- if(ar[i+1]==tw)
- {
- tr[i]=tr[i+1]+1;
- }
- else
- {
- tr[i]=tr[i+1];
- }
- }
- LL ans=0;
- FOR(i,n)
- {
- //cout<<ar[i]<<tr[i]<<endl;
- if(ar[i]==on)
- {
- ans+=tr[i];
- }
- }
- printf("%lld\n",ans);
- return 0;
- }
Problem 6:- Chef and confusing road
Tell you what Deja vu problem Unbearable Controversy of being. with relaxed constraints. My solution which got TLE on codeforces AC here on one go.
The procedure i followed was to take the any two nodes and find next successive members of the graph and take the intersection of both and add it to the count.
- #include <bits/stdc++.h>
- using namespace std;
- #define FOR(i,a) for(int i = 0;i < a;i++)
- #define REP(i,a,b) for(int i = a;i < b;i++)
- #define vi vector<int>
- vector<set<int> >adjlist;
- set<int>intersect;
- int main()
- {
- ios_base::sync_with_stdio(false);
- int m,n,a,b,drc=0;
- cin>>n>>m;
- adjlist.resize(n);
- FOR(i,m)
- {
- cin>>a>>b;
- adjlist[a-1].insert(b-1);
- }
- FOR(i,n)
- {
- for(set<int>::iterator it=adjlist[i].begin();it!=adjlist[i].end();it++)
- {
- int p1=*it,p2;
- set<int>::iterator it1=it;
- it1++;
- while(it1!=adjlist[i].end())
- {
- p2=*it1;
- intersect.clear();
- set_intersection(adjlist[p1].begin(),adjlist[p1].end(),adjlist[p2].begin(),adjlist[p2].end(),std::inserter(intersect,intersect.begin()));
- if(intersect.find(i)!=intersect.end())
- {
- drc+=(intersect.size()-1);
- }
- else
- {
- drc+=(intersect.size());
- }
- it1++;
- }
- }
- }
- cout<<drc<<endl;
- return 0;
- }
See you guys Later.
Adios.